Datatables is powerful but the documentation can be confusing in places. If you want a column to sort on a date one option (of many) is to have a formatted date to display to the user and an integer timestamp to use for the sorting.
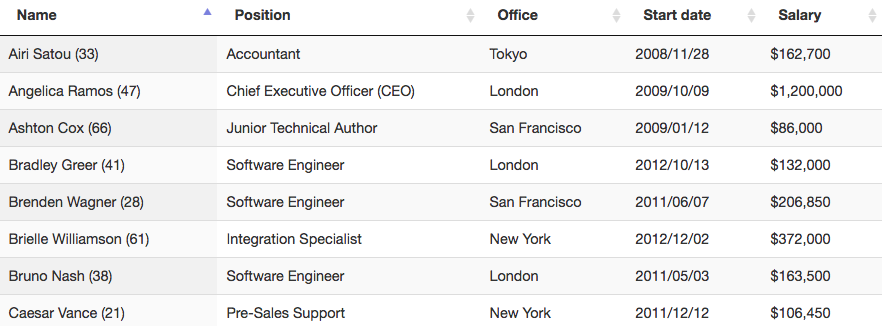
Here is some JSON that could be used to create this
var data = [
{
"name": "Airi Satou (33)",
"position": "Accountant",
"office": "Tokyo",
"displayStartDate": "23\/01\/03",
"startDate": "1043362800",
"salary": "162700",
},
{
"name": "Angelica Ramos (47)",
"position": "Chief Executive Officer (CEO)",
"office": "London",
"displayStartDate": "17\/01\/03",
"startDate": "1042761600",
"salary": "1200000",
}
];
The JavaScript used to get proper sorting of the date column involves adding an extra object to the date column which allows another data item to be used for the sort.
$('#example').DataTable( {
data: data,
columns: [
{ data: 'name' },
{ data: 'position' },
{ data: 'office' },
{ data: {
_: 'displayStartDate',
sort: 'startDate'
}},
{ data: 'salary'}
]
} );
One advantage of this approach is that date formatting is done up on the server which may be useful if you are sending tens of thousands of rows of data to the client.